Over the past few weeks, a number of users have informed us that they have encountered debugging a conditional C # assembly.
Approved: Fortect
In C #, go to the Build tab in the left pane of the property sheet, and then choose how to check the boxes in the compiler options. Delete the audit entries for the settings you want to disable. Place a conditional compiler switch on the call line. The compiler will include tracing, also known as debug code, into the executable.
- 12 minutes to read.
Although the compiler does not have a dedicated preprocessor, the directives described in this section are treated as if there was one. You use them to facilitate conditional collection. Unlike C C ++ and directives, you cannot use these directives to create macros. A preprocessor directive can only consist of one-line classes.
Nullable Context
Approved: Fortect
Fortect is the world's most popular and effective PC repair tool. It is trusted by millions of people to keep their systems running fast, smooth, and error-free. With its simple user interface and powerful scanning engine, Fortect quickly finds and fixes a broad range of Windows problems - from system instability and security issues to memory management and performance bottlenecks.
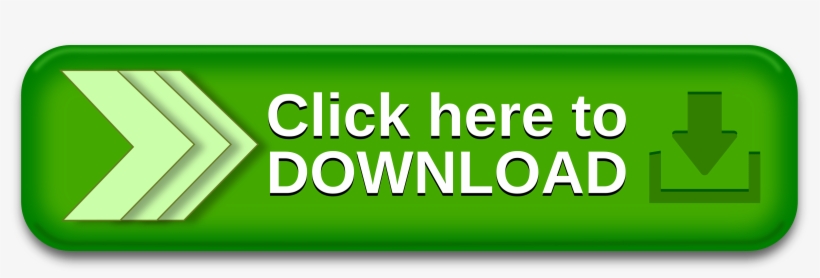
The #nullable
preprocessor information sets the nullable context annotation as the nullable alert context. This directive controls whether nullable rrf annotations are in effect and nullable notifications are issued. Each context is either disabled or enabled.
Both contexts can be specified as a source at the project level (outside of C # code). The #nullable
statement controls the entire context of annotations and warnings and takes precedence over project-level settings. A directive usually sets the context that controls it until another directive takes precedence over it, or until the end of that source file.
-
#nullable disable
: Disables warnings and nullable annotation contexts. -
#nullable enable
: Specifies a nullable annotation warning and the contexts to enable. -
#nullable restore
: restore nullable warning and annotation context in project settings. -
#nullable remove Annotations
: Sets the annotation context to be nullable to help you disable it. -
#nullable enable Annotations
: activates the annotation situation, omitting the NULL value. -
#nullable restore annotations
: restore our own nullable context annotation in project settings. -
# Eliminate Nullable Warnings
: Disable Nullable Context Warnings. -
#nullable warnings
: enable Enables a nullable warning. -
#nullable restore warnings
: restore the current nullable warnings in the context project settings.
Conditional Compilation
-
#if
: Opens conditional compilation, in which the code is compiled only if the specified symbol is almost certainly defined. -
#elif
: closes the previous conditional compilation and opens a new conditional compilation based on what is defined in the specified symbol. -
#else
: close before conditional compilation and open the resulting conditional compilation if no previously specified image is defined. -
#endif
: Close the previous dependent compilation.
If the C # compiler finds an observable #if
directive, perhaps using the #endif
directive, ideally it will compile the code between directives if specified. symbol is defined. Unlike C C ++, you cannot assign a numeric value to a character. The #if
statement in C # is boolean and only checks if a character is not specified or not. For example:
#if DEBUG Console .WriteLine ("Debug version");#end if
You can use the ==
(equality) and the skip ! = (inequality)
to query for bool
true
and false
values. true
means the symbol is defined. The #if DEBUG
statement has the same meaning as #if (DEBUG True)
==. You can use &&
(and) , <. use code> || (or) , but !
(not) operators to evaluate whether multiple characters are defined. sometimes group characters and operators with parentheses?
#if
, mostly with #else
, #elif
, #endif
, # define
and therefore the #undef
directives, you can includeAdd them depending on the presence of one or more symbols, or exclude coupons. Conditional compilation can be useful when compiling code for your debug version or compiling a single configuration.
A conditional start directive with any type of #if
directive must explicitly end with a different #endif
directive. With #define
you can define an important symbol. When using the use character as a specific expression passed to the #if
directive, your expression evaluates to true
. You can define the symbol using the DefineConstants parameter compiler. You can replace the symbol using the #undef
image. The size of the symbol depends on #define
with is the file it was defined in. The character a that you define with DefineConstants or #define
cannot conflict with a variable of the same name. A variable name must not be passed to a preprocessor directive, or a character can only be evaluated by a preprocessor directive process.
With
#elif
you can create a conditional A compound directive. The #elif
expression might very well evaluate if neither the previous #if
nor any of the optional preceding words and phrases of the #elif
directive are evaluated, become true
. If the name #elif
evaluates to true
, the compiler almost evaluates the code between the #elif
type of the next conditional directive. For example:
#define VC7// ...# when debugging Console.Build ");#elif Writeline ("debug VC7 Console.WriteLine ("Visual Studio 7");#end if
#else
allows anyone to create a compound conditional directive, so why if none of the expressions in the last #if
or (optional) # elif Compare
and comparing them to true
, the compiler evaluates every single code between #else
and the next #endif
. #endif
(#endif) should be the next command preprocessor after #else
.
#endif
indicates the end of any conditional directive starting with our own #if
directive.
The build system also pays attention to predefined preprocessor symbols that represent various target boardsforms in SDK style projects. They are useful when building applications targeting multiple versions of .NET.
Target frames | characters |
---|---|
.NET Framework | NETFRAMEWORK , NET48 , NET472 , NET471 , NET47 , NET461 , net462 , NET46 , NET452 , NET451 , NET45 , NET40 , NET35 , NET20 |
.NET standard | NETSTANDARD , N |