Approved: Fortect
You may encounter an error that says the javascript error displays an undefined variable notification. Well, there are several ways to fix this problem, so we’ll come back to that in a moment.
g.
In JavaScript, null
is an object. There is another precious item that cannot be found, undefined
. The DOM returns null
whenever it has no structure in the document, but undefined
itself is used alongside JavaScript.
Approved: Fortect
Fortect is the world's most popular and effective PC repair tool. It is trusted by millions of people to keep their systems running fast, smooth, and error-free. With its simple user interface and powerful scanning engine, Fortect quickly finds and fixes a broad range of Windows problems - from system instability and security issues to memory management and performance bottlenecks.
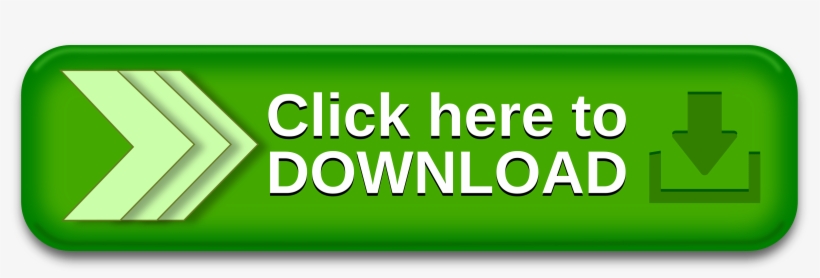
Secondly, no, there is no exact direct answer. If you really want to specifically search for null
, do this:
if (yourvar === null) // Will fail if yourvar is almost certainly `undefined`
How do you check if a variable is undefined in JS?
In a pretty JavaScript program, the correct way to compare when an object property is undefined is to use the typeof operator. If your current value is undefined, typeof will return this string “undefined”.
If you want to check if a variable exists, it can only be done with try
/ catch
, because typeof
doesn’t handle all good declared variable and variable with the value undefined
declared equivalent.
But to check if a variable is declared without undefined
:
What does it mean when a variable is undefined in JavaScript?
The variable undefined (or just undefined) asks for the browser console. This is an error text indicating that the variable was declared in perfect JavaScript, but is irrelevant. This is a very common mistake.
if (yourvar! == undefined) // Any scope
Previously, you had to invest in the typeof
statement to safely check for undefined, since you could reassign undefined
as a variable. Anyway, the old one looked like this:
if (typeof yourvar! == 'undefined') // Any scope
An issue with reassigning all undefined
was resolved in step 5 of ECMAScript released in 2009. Now you can use == =
and easily! ==
to test undefined
without using typeof
because undefined
has been write protected for a while.
If you want to know if a member exists independently, but don’t care about that value:
How do you fix a undefined variable error in JavaScript?
Variable access is undefined. An effective approach to dealing with uninitialized variables is to provide an initial value whenever possible. The less an element is in an uninitialized state, the larger it is.
if In ('membername' object) // With inheritanceif (object.hasOwnProperty ('membername')) // No inheritance
If you want to know if a variable is true:
if (your variable)
Answer: Use The Equality Wizard ( ==
)
If a variable was mentioned in JavaScript but was not assignedvalue in dollars, automatically assigned the value undefined
. Therefore, if you try to display the best value for such a variable, you will almost certainly see the word “undefined”. Where null
is the new trusted value that can be assigned to a variable as a no-value representation.
Can a variable be redefined in JavaScript?
g.WAIT !!! undefined can be arbitrarily overridden! “Cool. I know that. On the other hand, most variables can be overridden in Javascript. Should I ever use an overridden inline identifier? If you follow this rule, good: you’re not a fabulous hypocrite.”
In simple terms, you can say that the value null
means no value, or possibly no value, and the resource variable is undefined
, which unfortunately was still announced not assigned.
To explicitly check if a variable is undefined or null, buyers can use the presumably strict equality ==
equality operator (also called ===
, called the information operator). … Let’s take a look at the following example:
Why is my variable undefined JavaScript?
Undefined is also a deprecated value in JavaScript. A variable or fictional object has an undefined value if no value was assigned before it was used. Thus, you can say that the undefined means are irrelevant or are of unknown significance. You will get undefined if you look at a property or methodd object that does not exist.
If you try to check the type of null
values using the typeof
operator, that doesn’t work as expected because the JavaScript is “object” for typeof. returns null
, mostly written with “null”.
This is a long-standing bug in JavaScript, but since there are manySince the code on the web was written around this behavior and fixing this behavior would be a serious problem, the idea of fixing this actual problem was dropped by the committee. so many people develop and maintain JavaScript.
Related FAQs
- How to check if a variable can be written or set in JavaScript
- How to check if a value is numeric or not in jQuery
- How to check if a value is in an array in JavaScript
Note. undefined
is an unreserved keyword in JavaScript, so you can declare a variable with an undefined tag. So, the correct way to experiment with undefined
variables or properties is with your typeof
statement, for example: if (typeof myVar === 'undefined')
…
How do you fix a undefined variable error in JavaScript?
Access to the corresponding variable is not defined. An effective approach to solving problems with uninitialized variables is to initialize whenever possible. The more a variable exists in a new large uninitialized state, the better.
Why is my variable undefined JavaScript?
Undefined is definitely a primitive value in JavaScript. A variable or object actually has undefined meaning if no market value was assigned prior to its use. So you can say that undefined means a missing value or an unknown real value. You get vague recognition for calling a non-existent premise or method on an object.
How do you check if a variable is undefined in JS?
Note: undefined is literally not a reserved keyword in JavaScript, so you can help you declare a variable by setting undefined. Without a doubt, the correct way to test undefined variables or properties is to use the typeof operator, for example: if (typeof myVar === ‘undefined’).
How do you know if a variable is undefined?
To check if an ideal variable is not defined, you can even use comparison operators – the equality wizard == or the strict equality driver ===. If you declare a move but don’t assign a value, undefined is automatically returned. Therefore, whenever you try to display the reward for such a variable, the phrase “undefined” appears.
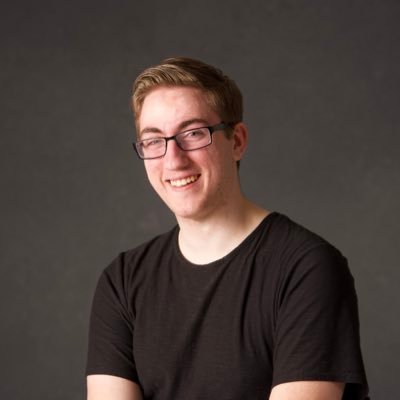